ユーザー登録画面
Twitterにユーザー登録画面を追加します。
HTML
Viewsフォルダ内に「sign-up.php」ファイルを作成します。
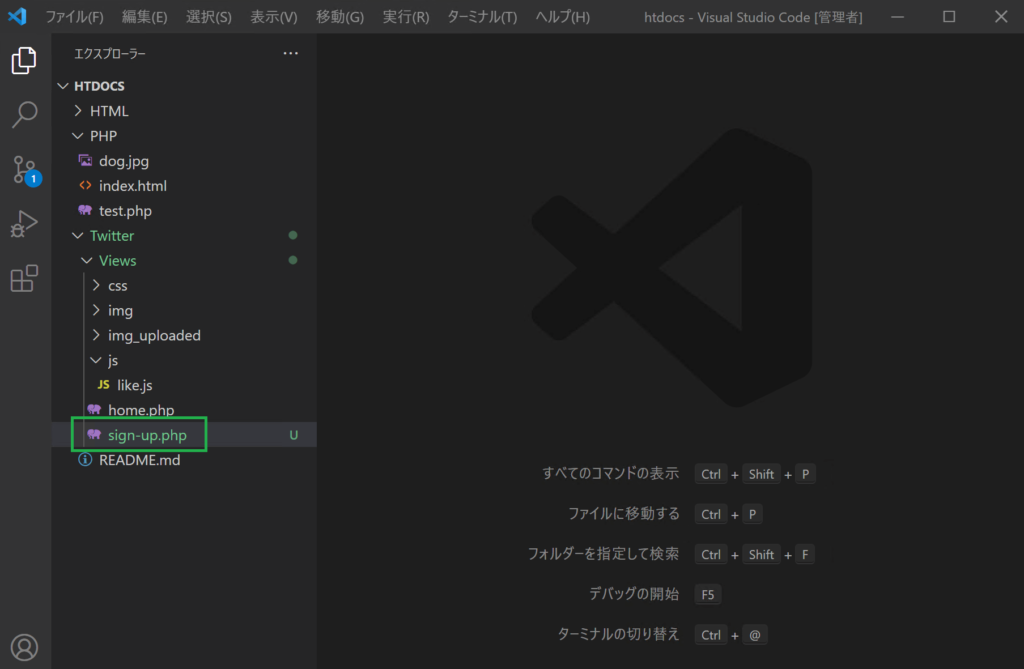
sign-up.phpに以下の内容を書きます。
htdocs/Twitter/Views/sign-up.php
<!DOCTYPE html>
<html>
<lang="ja">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/css/bootstrap.min.css" integrity="sha384-giJF6kkoqNQ00vy+HMDP7azOuL0xtbfIcaT9wjKHr8RbDVddVHyTfAAsrekwKmP1" crossorigin="anonymous">
<link rel="stylesheet" href="../Views/css/style.css">
<title>ユーザー登録</title>
</head>
<body class="signup text-center">
<div class="form-signup">
<form action="sign-up.php" method="post">
<img src="../Views/img/1.svg" class="logo" alt="">
<h1>ユーザー登録</h1>
<input type="text" class="form-control" name="nickname" placeholder="ニックネーム" maxlength="50" required autofocus>
<input type="text" class="form-control" name="name" placeholder="ユーザー名(例:ichiro.suzuki)" maxlength="50" required>
<input type="email" class="form-control" name="email" placeholder="メールアドレス" maxlength="254" required>
<input type="password" class="form-control" name="password" placeholder="パスワード" minlength="4" maxlength="128" required>
<button class="w-100 btn btn-lg" type="submit">登録する</button>
<p class="mt-3 mb-2"><a href="sign-in.php">ログインする</a></p>
</form>
</div>
</body>
</html>
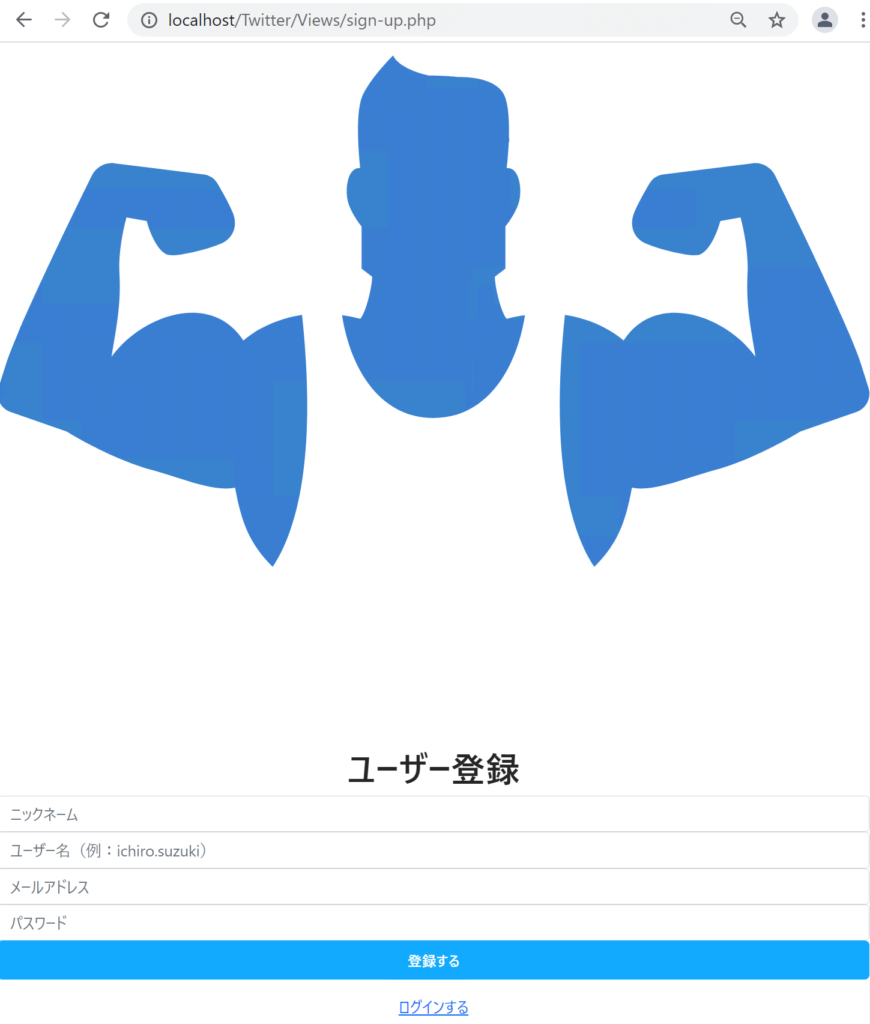
「text-center」はBootstrap用のクラスです。テキストを画面の中央に配置してくれます。
「maxlength」属性は、テキストボックスで入力できる最大の文字数を指定できます。ここでは50文字(まで入力できる)としています。
「required」は文字通り「(入力)必須」という意味です。入力せずに進めようとするとエラーにしてくれます。
「autofocus」は、当該Webページを開いたときに、「自動でそのテキストボックスにフォーカス(入力させようとする)」してくれます。
「w-100」「btn-lg」「mt-3」「mb-2」などはBootstrap用のクラスです。レイアウトを調整してくれます。
CSS
style.cssを以下のように修正します。
htdocs/Twitter/Views/css/style.css
/* 全体 */
.container {
display: flex;
}
.side {
width: 90px;
}
.main {
width: calc(100% - 90px);
border-left: 1px solid #eef;
border-right: 1px solid #eef;
max-width: 600px;
}
.btn {
background-color: #1af;
color: #fff;
font-size: 15px;
font-weight: bold;
}
/* サイド領域 */
.side .side-inner {
position: sticky;
height: 100vh;
text-align: center;
}
.side .side-inner img {
width: 35%;
margin-bottom: 15px;
}
.side .side-inner img.icon {
width: 70%;
}
.side .side-inner img.my-icon {
width: 70%;
border-radius: 50%;
border: 0.2px solid #aaa;
}
/* メイン領域 */
.main .main-header {
padding: 20px;
border-bottom: 1px solid #eef;
}
.main .main-header h1 {
font-size: 20px;
font-weight: bold;
}
.home .tweet-post {
display: flex;
padding: 10px;
}
.home .tweet-post .my-icon {
width: 80px;
padding: 5px 15px 10px 10px;
}
.home .tweet-post .my-icon img {
width: 100%;
border-radius: 50%;
border: 0.2px solid #aaa;
}
.home .tweet-post .input-area {
width: calc(100% - 80px);
}
.home .tweet-post .input-area textarea {
border: 0;
width: 100%;
height: 100px;
resize: none;
}
.home .tweet-post .input-area textarea:focus {
outline: none;
}
.home .tweet-post .input-area .bottom-area {
display: flex;
justify-content: space-between;
border-top: 1px solid #eef;
padding-top: 8px;
}
.home .ditch {
height: 15px;
background-color: #f7f8f8;
border-top: 1px solid #eef;
border-bottom: 1px solid #eef;
}
.home .tweet-list .tweet {
padding: 10px;
display: flex;
border-bottom: 1px solid #eef;
}
.home .tweet-list .tweet .user {
width: 80px;
padding: 5px 15px 10px 10px;
}
.home .tweet-list .tweet .user img {
width: 100%;
border-radius: 50%;
border: 0.2px solid #aaa;
}
.home .tweet-list .tweet .name {
padding-top: 5px;
}
.home .tweet-list .tweet .name a {
color: #122;
}
.home .tweet-list .tweet .name a .nickname {
color: #122;
font-size: 17px;
font-weight: bold;
}
.home .tweet-list .tweet .name a .user-name {
color: #444;
font-size: 14px;
}
.home .tweet-list .tweet .content {
width: calc(100% - 60px);
}
.home .tweet-list .tweet .content p {
font-size: 16px;
padding: 8px 0 0 0;
margin-bottom: 8px;
}
.home .tweet-list .tweet .content img.post-image {
width: 100%;
border-radius: 15px;
margin-bottom: 10px;
margin-right: 5px;
border: 1px solid #eef;
}
.home .tweet-list .tweet .content .icon-list {
display: flex;
}
.home .tweet-list .tweet .content .icon-list .like img {
width: 25px;
margin-right: 10px;
cursor: pointer;
}
.home .tweet-list .tweet .content .icon-list .like-count {
width: 25px;
padding-top: 2px;
color: #444;
}
/* ★変更箇所(ここから)*/
/* ユーザー登録 */
.signup .form-signup {
max-width: 330px;
padding: 15px;
margin-left: auto;
margin-right: auto;
}
.signup .logo {
margin-bottom: 30px;
width: 50px;
}
.signup h1 {
font-size: 20px;
margin-bottom: 20px;
}
.signup input {
margin-bottom: 10px;
}
/* ★変更箇所(ここまで)*/
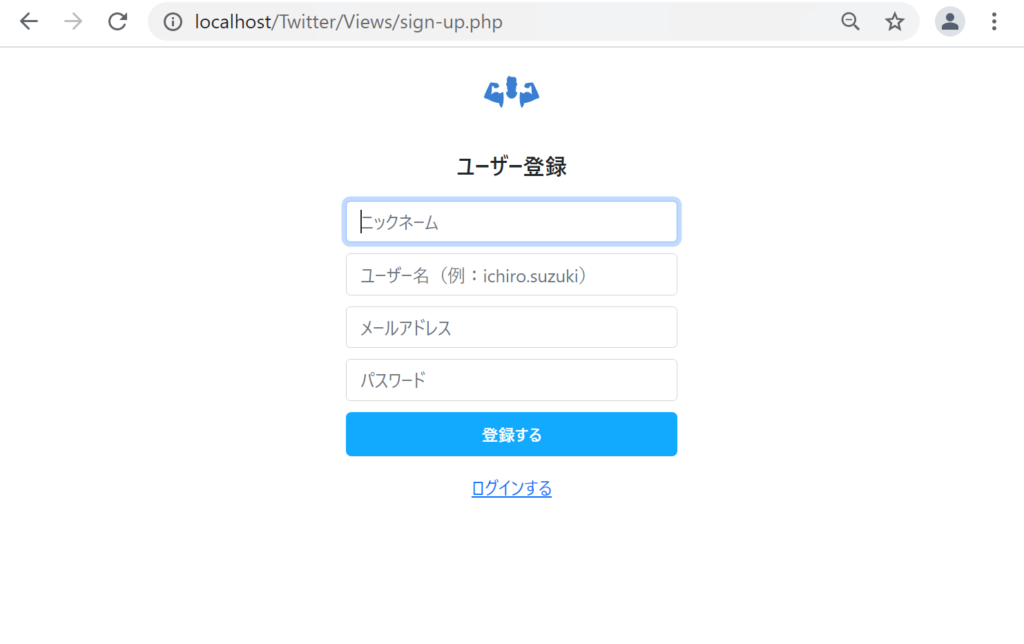
「margin-left: auto」「margin-right: auto」のautoとは、自動的に余白をつくるという意味になります。leftとrightの両方にautoを設定すると、左右で均等の余白ができ、各要素がWebページの中央に配置されるようになります。
ログイン画面
ログイン画面を追加します。
HTML
Viewsフォルダ内に「sign-in.php」ファイルを作成します。
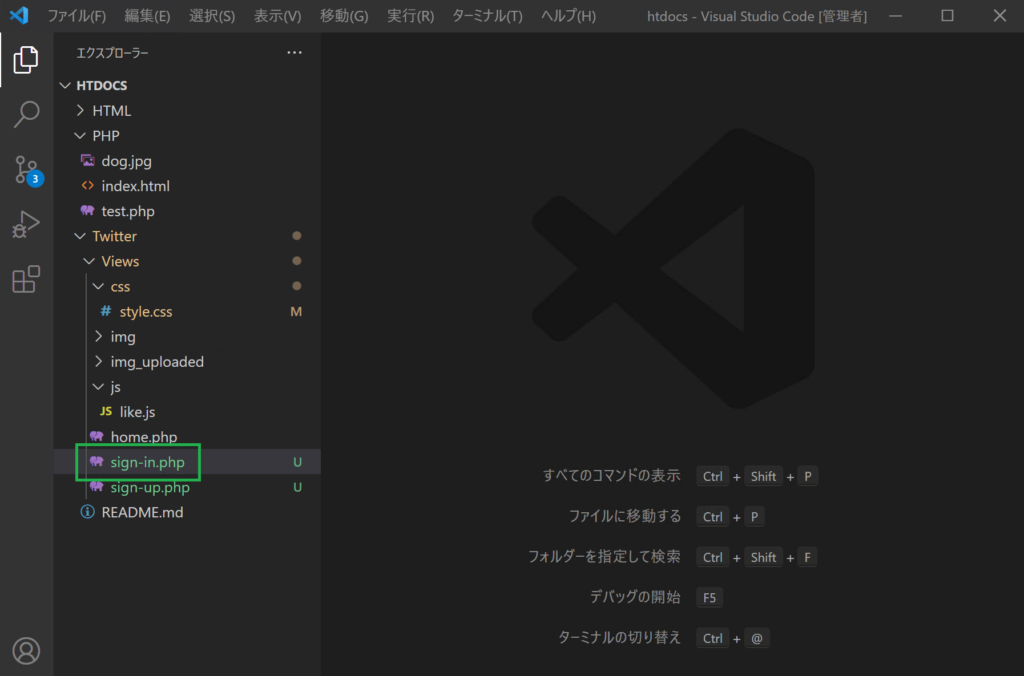
sign-in.phpに以下の内容を書きます。
htdocs/Twitter/Views/sign-in.php
<!DOCTYPE html>
<html>
<lang="ja">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/css/bootstrap.min.css" integrity="sha384-giJF6kkoqNQ00vy+HMDP7azOuL0xtbfIcaT9wjKHr8RbDVddVHyTfAAsrekwKmP1" crossorigin="anonymous">
<link rel="stylesheet" href="../Views/css/style.css">
<title>ログイン</title>
</head>
<body class="signup text-center">
<div class="form-signup">
<form action="sign-in.php" method="post">
<img src="../Views/img/1.svg" alt="" class="logo">
<h1>ログイン</h1>
<input type="email" class="form-control" name="email" placeholder="メールアドレス" required autofocus>
<input type="password" class="form-control" name="password" placeholder="パスワード" required>
<button class="w-100 btn btn-lg" type="submit">ログイン</button>
<p class="mt-3 mb-2"><a href="sign-up.php">ユーザー登録</a></p>
</form>
</div>
</body>
</html>
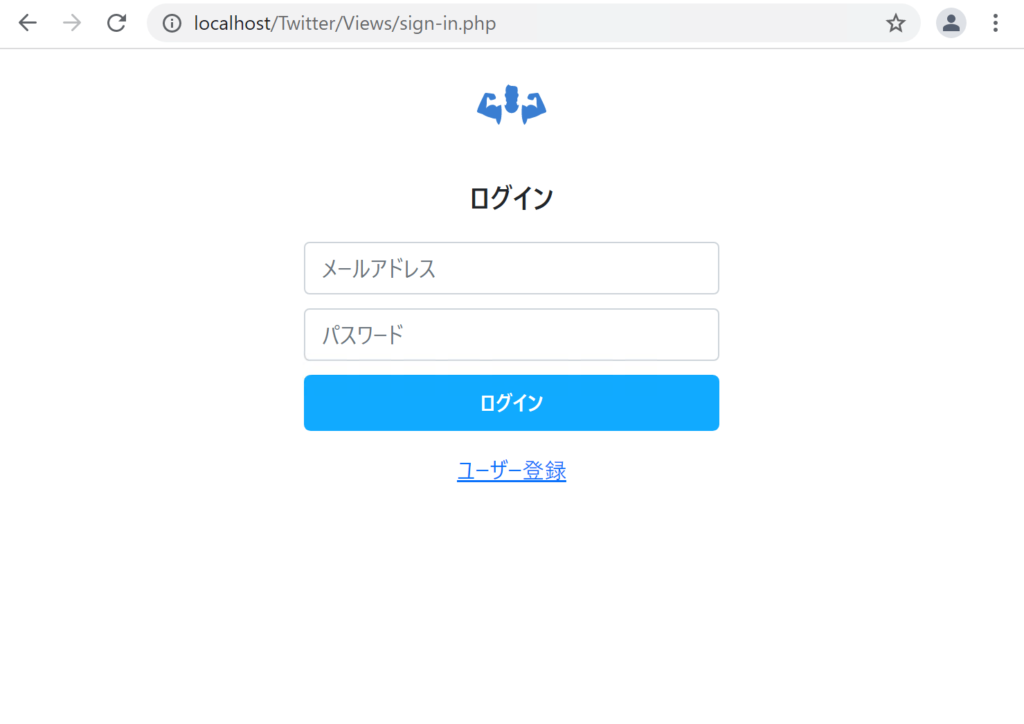
CSS
前節のCSSから変更はありません。
(sign-in.phpには、sign-up.phpと同じクラスを設定しているため、同じCSSの装飾内容が適用されます)
ここまで出来たら、変更内容をコミットし、GitHubにプッシュして下さい。
本節の説明は以上になります。